How to use the while-Loop in Java
With the while-loop in Java, you can execute statements until a termination condition occurs. This loop form can also be combined and nested.
- 99.9% uptime
- PHP 8.3 with JIT compiler
- SSL, DDoS protection, and backups
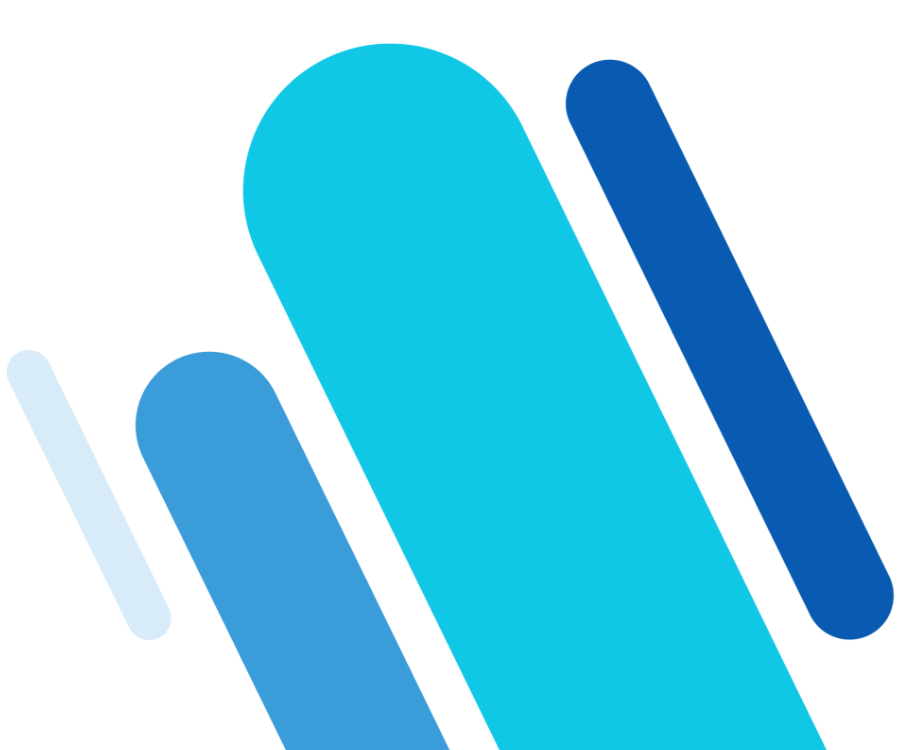
What is the while-loop for Java?
The while-loop provides Java users with the ability to execute any number of statements as long as a previously defined condition is true. A count variable is not required, so the termination condition can also be defined separately.
The termination condition works after the Boolean. This means that the while-loop in Java executes the stored statements as long as the condition specified in the header is true. If the termination condition changes to “false” for the first time, the loop is terminated. Because the termination condition is already set in the head of the while-loop in Java, it is called a head-controlled loop.
How is the while-loop constructed in Java?
The structure of a while-loop in Java doesn’t usually change too much. While the syntax remains the same, only the statements and the termination condition change. First the term “while” introduces the loop, then the termination condition follows in brackets and finally one or more statements in curly brackets. This is what a while-loop looks like in Java source code:
while ( termination condition) {
Statement
}
The flow always follows this order:
- The program checks the condition.
- If it is met, the Java application executes the statement.
- Then the program jumps back to the beginning.
- Again, the condition is checked.
- If it is now “false”, the program exits the while-loop in Java and continues through the code.
Example of a simple loop
A simple example of a while-loop in Java is a counter that counts up to a certain value. It does this exactly until the specified value (the termination condition) is reached. Written out, it looks like this:
int i = 0;
while ( i <= 5 ) {
System.out.println ( "The current value is at: " + i );
i++;
}
The variable “i” starts at zero in this setup. It should now increase by 1 until it reaches the value “5”. This is done by the Java operator ++. In the next round, when the value would be “6”, the program terminates and the while-loop in Java is terminated. This is indicated by the Java command System.out.println the number series 1, 2, 3, 4, 5.
Avoiding endless loops
Although the while-loop in Java is simple in structure, it does have a few potential error sources that can cause the loop fail. If an important piece of information is missing or the termination condition cannot be fulfilled, you’ll get an infinite loop. This either causes a crash or stops the program at some point. This happens, for example, if the check of the termination condition always returns “true” or the targeted value cannot be reached.
The difference between while and do-while in Java
In addition to the while-loop in Java, there is also the do-while-loop. This not only has a similar name, but also works almost exactly like the while-loop. The main difference is that the head-controlled while-loop checks the termination condition in advance, and the foot-controlled do-while-loop waits until the statements have been executed at least once.
This can be seen in the following structure:
int i = 0;
do {
System.out.println ( "The current value is at: " + i );
i++;
}
while ( i <= 5 );
Here, the code is executed at least once and then the condition is checked afterwards.
Nesting while-loops in Java
You also have the option of nesting several while-loops in Java. An example with two counters looks like this:
int i = 0;
while ( i
System.out.println ( "The current value is: " + i );
i++;
int Intermediate step = 0;
while ( intermediate step <= 3 ) {
System.out.println ( "The current intermediate value is at: " + intermediate step );
IntermediateStep++;
}
}
So, first the outer while-loop is checked by the Java program. If it is “true”, the program jumps to the inner loop and checks it as well. Now the inner loop is checked until its termination condition is “false”. Then the program switches back to the outer loop.
The difference between for and while in Java
Besides the while-loop for Java presented here and the do-while-loop also taken up, there is also the for-loop. This resembles the Java while-loop at first sight, since it too repeats a code until the defined termination condition becomes “false”. Unlike the while-loop, however, the for loop still has a run variable that is initialized before the termination condition.