Less CSS Tutorial
Anyone who wishes to program or design modern websites will not be able to avoid CSS. The stylesheet language is – like HTML – one of the core languages of the World Wide Web and enables website content to be clearly distinguished from its graphical presentation. This allows variables like layout, colors, or typography to be adjusted at any time, without having to completely overhaul the source code. This design-related information is stored in stylesheets based on CSS. However, the larger a web project is, the more complex and confusing stylesheets become – and hence the more difficult it becomes to work with the web language. The CSS preprocessor Less provides welcome relief here.
What is Less?
Less (Leaner Style Sheets) is a reverse-compatible language extension or preprocessor for the stylesheet language CSS. This means that any CSS code is also automatically a valid Less code (but this is not true in the other direction). The purpose of Less is to make writing CSS code more efficient. The language influenced by SASS thus offers a range of additions to the standard CSS instructions, including variables and functions that, for instance, enable easier stylesheets and eliminate the need for tedious code duplication.
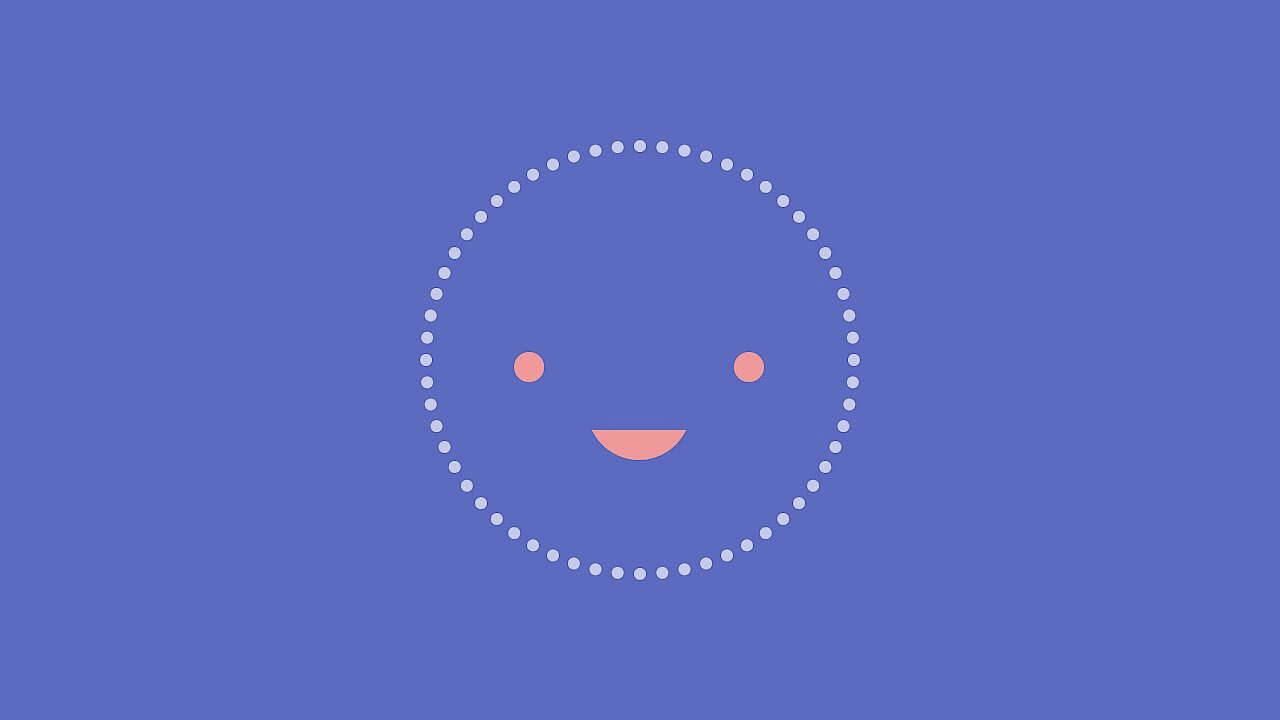
When Alexis Sellier released the CSS preprocessor in 2009, the compiler for converting Less into CSS was still written in the object-based programming language Ruby. Now, however, the JavaScript tool Less.js forms the basis of the stylesheet language and the compilation process – with the advantage that Less cannot only be compiled on the server side but also on the client side (in web browsers).
Less vs. CSS: How do they differ?
Both CSS and Less are considered to be stylesheet languages. They are hence formal languages that determine the appearance of user interfaces and documents. The stylesheet files containing the desired design instructions simply need to be assigned to the HTML elements of a website; the browser takes care of interpretation. The key difference between Less and CSS is that CSS is a static language, whereas Less ranks among the dynamic languages and therefore also offers dynamic features such as variables, operators, functions, mixins, and nesting that are not available in CSS.
While instructions in CSS have to follow a fixed scheme, Less opens up substantially more flexible possibilities for developers: For example, individual rules can be defined for any class of elements throughout an entire stylesheet – rendering tedious code repetition unnecessary. As a result, the syntax of the two languages also varies. Less syntax can generally be classified as a metasyntax of CSS, as valid CSS code is also always valid Less code with the same semantics.
How to use Less on clients and servers
If you’d like to use Less for your project, you have two options: You can either use the web browser of your choice to compile your Less stylesheets on the client side via Less.js, or you can install the JavaScript application on your development computer and convert your code there using Less.js and the JavaScript runtime environment Node.js via command line.
Less CSS: Use on clients
Using Less within a client is the easiest and quickest way to work with the stylesheet language. But this method is not recommended for the subsequent live environment, since the additional compilation of Less to CSS would result in clear performance setbacks for accessing users. Moreover, browsers with disabled JavaScript would completely ignore the design instructions.
To compile Less in the browser, first specify in the corresponding document that you wish to use Less stylesheets (i.e. stylesheets with the file ending .less). Simply integrate them using the rel attribute “stylesheet/less”:
<link rel="stylesheet/less" type="text/css" href="styles.less">
Next, download the current version of Less.js – available for example in the official GitHub repository for the CSS preprocessor. You can then integrate the JavaScript tool in the <head> section of your project:
<script src="less.js" type="text/javascript"></script>
It’s important to first integrate the stylesheet and then the script. Otherwise, you may encounter processing issues.
Use of Less CSS on servers
Less is also quick to install and easily executed on development computers. Here, you have free choice in terms of the operating system: The CSS preprocessor runs on Windows, macOS, and UNIX/Linux. However, the above-mentioned JavaScript runtime environment Node.js has to be installed.
Start by downloading the current version for your system from the Node.js website and installing it. Using npm – the packet manager for the JavaScript runtime environment – you should then install the stylesheet language via command line:
npm install -g less
You can now compile prepared Less stylesheets at any time – likewise via the command line. The example file example.less can be converted into a CSS file, for example, with the following command:
lessc example.less example.css
Less tutorial: The main features of Less explained with examples
For anyone who regularly works with CSS, getting to grips with Less is well worth it. Using Less not only offers the advantage of being able to include dynamic behavior in stylesheet code, but also the ability to save time and effort. Here, however, it’s important to become familiar with how the CSS extension works. After all, to write stylesheets in Less, you also need to know the necessary syntax rules. Using practical Less CSS examples, our short Less tutorial introduces you to the most important features, including the corresponding notation.
Variables
One of Less’ biggest strengths is the option – as with other programming languages – to create variables. These can store any types of value and are especially relevant for values that you use very frequently in your stylesheet: For instance, variables are often used to centrally define colors, fonts, dimensions (size, height, and width), selectors or URLs as well as their variations (such as lighter/darker). The defined values can then be used anywhere in the stylesheet, allowing global changes to be implemented with just a single line of code.
The follow code extracts define two variables as examples – one for the background color (@background-color) and one for the text color (@text-color). Both contain hexadecimal codes:
// Less
@background-color: #ffffff;
@text-color: #000000;
p{
background-color: @background-color;
color: @text-color;
padding: 15px;
}
ul{
background-color: @background-color;
}
li{
color: @text-color;
}
The background color – in this case white – is assigned to both common text blocks (p) as well as unordered lists (ul). Black is set as the text color and applies to text in text blocks as well as for list elements (li) in lists. To perform changes to these defined colors and specify, for example, white text on a black background for lists and text passages, all you need to do is exchange the values of the two variables. In a standard CSS sheet, the values would have to be replaced individually for all elements. After compiling into CSS, the code then appears as follows:
/* CSS */
p{
background-color: #ffffff;
color: #000000;
padding: 15px;
}
ul{
background-color: #ffffff;
}
li{
color: #1A237E;
}
Mixins
Mixins are similar to variables. But in this case, it’s not individual values but whole classes as well as the values specified therein that are centrally defined in order to then be passed on at any time to other classes in the Less stylesheet. Mixins can also behave like functions and accept parameters (including default values). The following example is a mixin for rounded corners (.rounded-corners) that is assigned to both the header (#header) and footer (#footer). While the specified value is accepted for the header, #footer gives the mixin its own value (10px):
// Less
.rounded-corners (@radius: 5px) {
border-radius: @radius;
-webkit-border-radius: @radius;
-moz-border-radius: @radius;
}
#header {
.rounded-corners;
}
#footer {
.rounded-corners(10px);
}
The compiled CSS for this Less code looks like the following:
/* CSS */
#header {
border-radius: 5px;
-webkit-border-radius: 5px;
-moz-border-radius: 5px;
}
#footer {
border-radius: 10px;
-webkit-border-radius: 10px;
-moz-border-radius: 10px;
}
Nesting
To create inherited attributes in CSS, you need to write long, complicated selectors. In Less, you can nest as many selectors within each other as you wish. This alleviates the work and also creates a clearer, more comprehensible structure in the stylesheet. This feature of the CSS preprocessor can also be illustrated with an example:
// Less
#header {
h1 {
font-size: 26px;
font-weight: bold;
}
p {
font-size: 12px;
a {
text-decoration: none;
&:hover {
border-width: 1px
}
}
}
}
The selectors p, a, and :hover are here bundled in the Less stylesheet, which greatly simplifies attribution in a CSS stylesheet. This is clear when looking at the generated CSS for this code example:
/* CSS */
#header h1 {
font-size: 26px;
font-weight: bold;
}
#header p {
font-size: 12px;
}
#header p a {
text-decoration: none;
}
#header p a:hover {
border-width: 1px;
}
Operators
The arithmetic operators addition (+), subtraction (-), multiplication (*), and division (/) can also be used in Less stylesheets by applying the respective operator to any number or color value. This means you can easily create complex interdependencies between the values of various elements which persist even when you change the base values. If conversion is rendered impossible or inappropriate by an operator, it will be automatically ignored – for example, if a centimeter value is added to a pixel value. The following example shows you how operators can be used in Less:
// Less
@the-border: 1px;
@base-color: #111;
#header {
color: (@base-color * 3);
border-left: @the-border;
border-right: (@the-border * 2);
}
#footer {
color: (@base-color + #003300);
}
The basic definitions for borders (1px) and the base color (#111), which corresponds to a black, are also applied to the header and footer, whereby three operators affect the base values:
- The base color is multiplied by three in the header. This results in the hex value #333, which equates to a dark gray.
- The right border in the header is given the multiplication operator * 2 and is therefore twice as wide as the standard border (2px).
- The basic color of the footer is likewise manipulated by an operator. Here, the hex value #003300 is added to the base value #111, which gives the footer a dark green (#114411).
The impressive results can also be seen in the compiled CSS code:
/* CSS */
#header {
color: #333;
border-left: 1px;
border-right: 2px;
}
#footer {
color: #114411;
}
Functions
Less also adds functions to CSS which provide a whole range of options: For example, you can add complex logical relationships with the if or Boolean function in a Less stylesheet. Or you can use functions that are no less complex for mathematical calculations such as cosine, sine, and tangent. On the other hand, basic functions for quickly defining colors (rgb, rgba, hsv, etc.) or functions for color operators such as contrast, saturate/desaturate or lighten/darken are also possible. To increase or decrease the saturation of an element, for instance, you only need a color value and the function saturate. By specifying a percentage (0–100%), you can also define the degree to which saturation should be changed.
// Less
@red: #842210;
#header {
color: saturate(@red, 20%); ->#931801
}
#footer {
color: desaturate(@red, 10%); ->##7d2717
}
In this example, the dark red color is defined with the hexadecimal code #842210 in the Less stylesheet and is entered as the color for the header and footer. But an increase of 20 percent is to be applied to the header, while the Less code should reduce the saturation in the footer by 10 percent. In the CSS stylesheet, the functions and the color variable (@red) are converted, and therefore only the hex codes with the respective saturation level can be seen:
/* CSS */
#header {
color: #931801
}
#footer {
color: #7d2717
}
Less CSS: Less work, more possibilities
This brief Less tutorial only touched on a fraction of the options that make the CSS preprocessor so useful. Once you have defined variables, mixins, and other functions, you can apply them to new elements in your stylesheet at any time – without starting again from scratch as is often the case with CSS code. If values such as the base color change, you can easily adjust them with little effort in a working Less document – which also makes the CSS preprocessor a valuable aid for the long-term presentation of a web project.
Detailed information on the individual Less features can be found in the in-depth guide on lesscss.org.